ProbityPeople uses a token-based API authentication.
An API Token contains your security credentials for the ProbityPeople platform. Generating an API Token allows you to access (i.e., make requests to) the ProbityPeople REST API upon successful authentication.
A user can create up to five API tokens. When creating a token, you must give it a description to help you remember what you created the token for.
API Token security
For security, you should regularly review the list of API tokens you have created and delete those that you no longer need.
Remember to keep your tokens secret and treat them just like passwords. Your tokens act on your behalf when interacting with the API. As such, do not hardcode them into your programs. Instead, opt to use them as environment variables.
API Token attribution
If you generate an API token from your ProbityPeople account, any API actions that are authenticated with your token will be attributed to you in the ProbityPeople portal. For example, if an API request uses your token to authenticate an API request to create a new order, the order will appear to be created by you in the ProbityPeople portal.
Generating an API Token
You can generate an API token from the settings->API page in the ProbityPeople business portal.
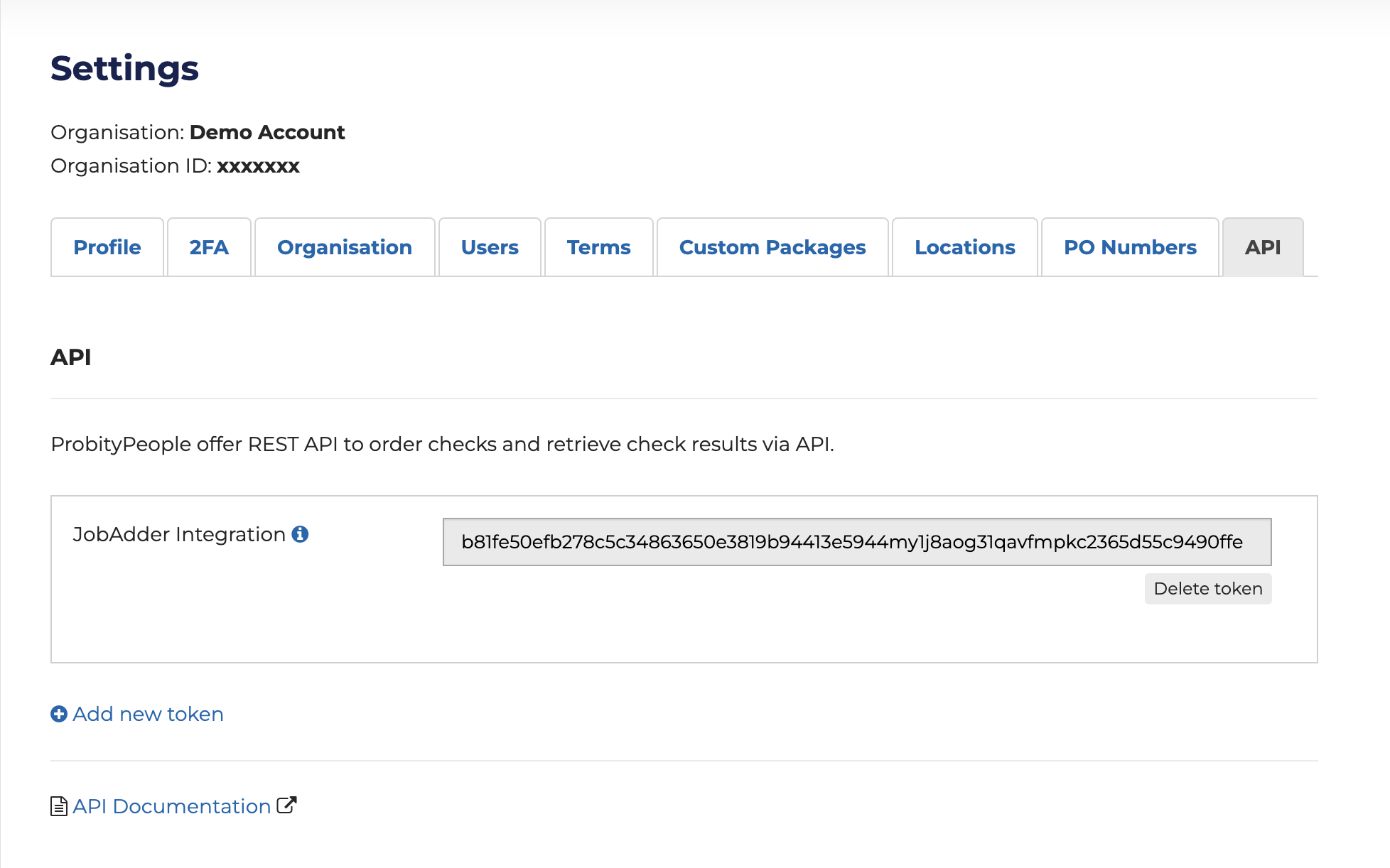
ProbityPeople - API setting page
Authenticating HTTP Requests
API tokens should be used similarly to OAuth access tokens when accessing the API (i.e., passing them in the Authorization header). HTTP requests can be authenticated with following two methods.
1. Authenticating with basic authentication
The API token will become the username of your request and the password can be left blank.
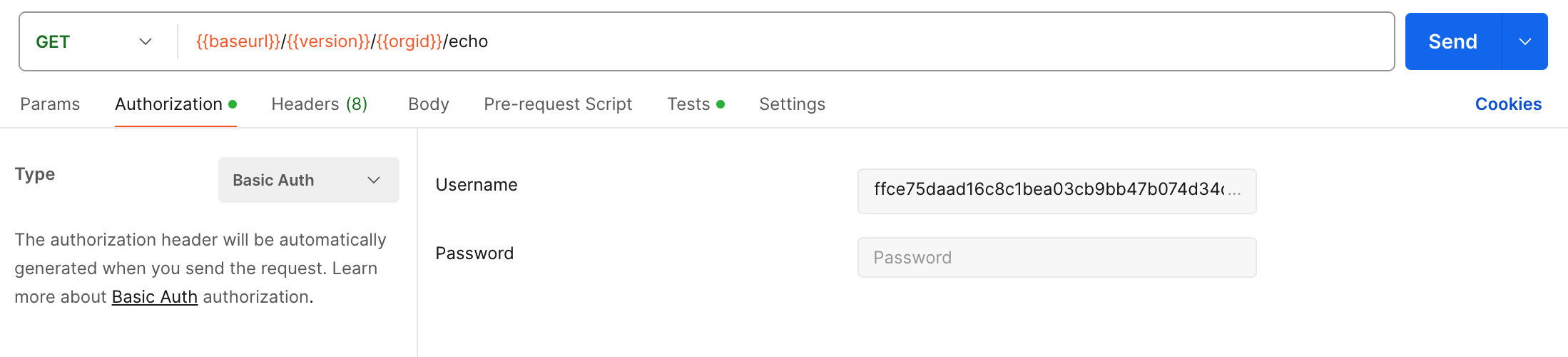
Postman - authenticating request with Basic Auth
2. Authenticating with bearer token
API token can also be passed on Authorization header as a Bearer token.
curl <API_ENDPOINT>
-H "Authorization: Bearer <YOUR_API_TOKEN>"
import requests
url = "<API_ENDPOINT>"
headers = {
"accept": "application/json",
"Authorization": "Bearer <YOUR_API_TOKEN>"
}
response = requests.get(url, headers=headers)
print(response.text)
const options = {
method: 'GET',
headers: {accept: 'application/json', Authorization: 'Bearer <YOUR_API_TOKEN>'}
};
fetch('<API_ENDPOINT>', options)
.then(response => response.json())
.then(response => console.log(response))
.catch(err => console.error(err));
$client = new \GuzzleHttp\Client();
$response = $client->request('GET', '<API_ENDPOINT>', [
'headers' => [
'Authorization' => 'Bearer <YOUR_API_TOKEN>',
'accept' => 'application/json',
],
]);
echo $response->getBody();
require 'uri'
require 'net/http'
url = URI("<API_ENDPOINT>")
http = Net::HTTP.new(url.host, url.port)
http.use_ssl = true
request = Net::HTTP::Get.new(url)
request["accept"] = 'application/json'
request["Authorization"] = 'Bearer <YOUR_API_TOKEN>'
response = http.request(request)
puts response.read_body
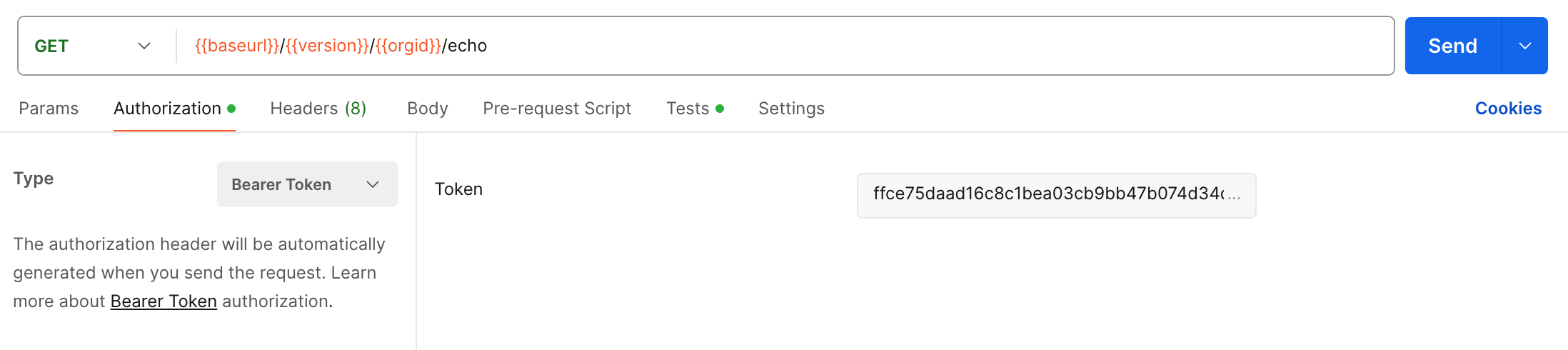
Postman - authenticating request with Bearer Token